Welcome to Milvus Docs!
Here you will learn about what Milvus is, and how to install, use, and deploy Milvus to build an application according to your business need.
Try Managed Milvus For Free!
Zilliz Cloud is hassle-free, powered by Milvus and 10x faster.
Get Started
Install Milvus
Learn how to install Milvus using either Docker Compose or on Kubernetes.
Quick Start
Learn how to quickly run Milvus with sample code.
Bootcamp
Learn how to build vector similarity search applications with Milvus.
Recommended articles
Deploy
What’s new in docs
June 2025 - Milvus 2.6.0 release
- Added guidance on how to use embedding function.
- Added guidance on how to use decay ranker.
- Added guidance on how to add fields to an existing collection.
- Added guidance on how to perform phrase match.
- Added descriptions of IVF_RABITQ index.
Blog
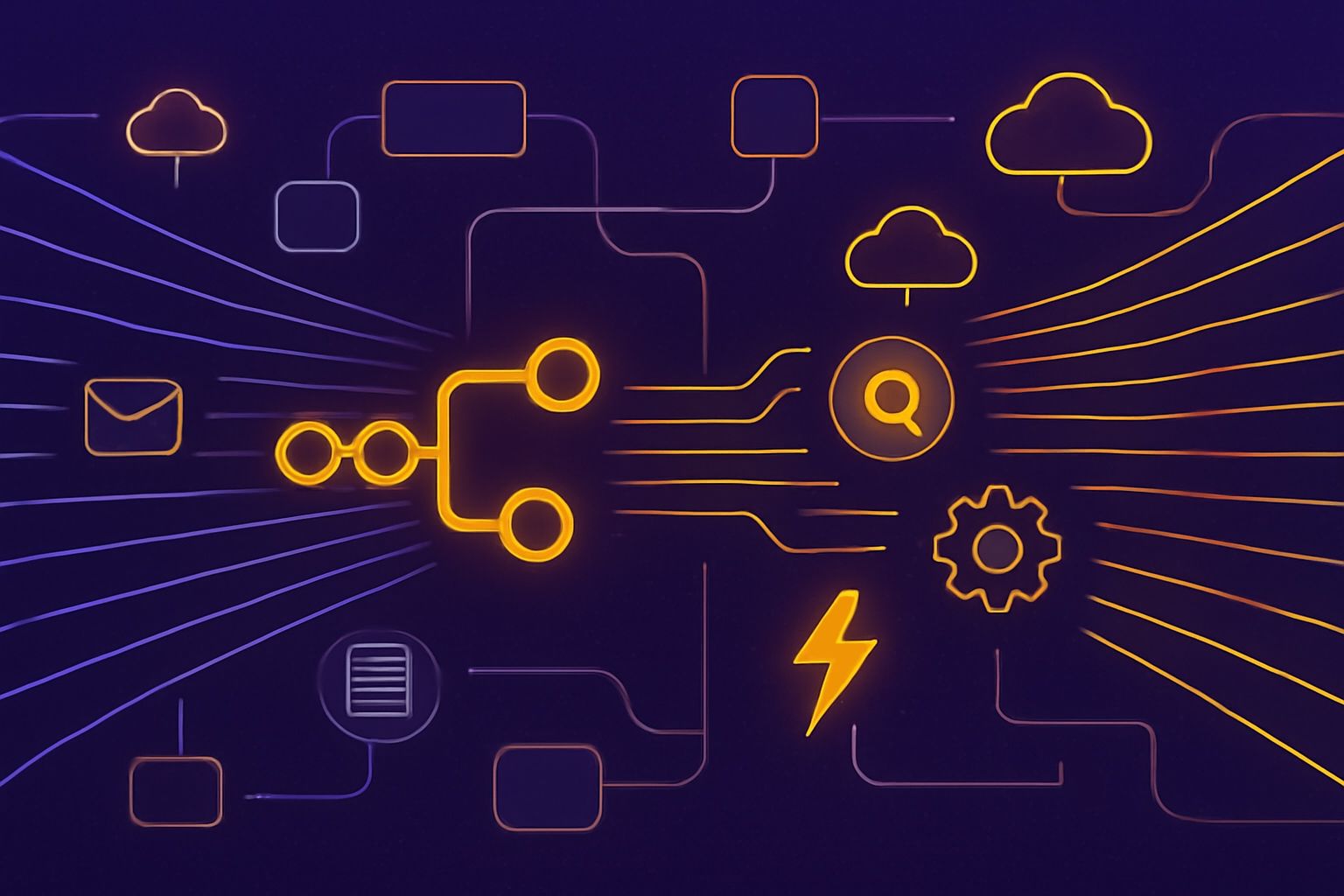
Engineering
I Discovered This N8N Repo That Actually 10x'd My Workflow Automation Efficiency
Learn how to automate workflows with N8N. This step-by-step tutorial covers setup, 2000+ templates, and integrations to boost productivity and streamline tasks.
Use